Real-Time Backend
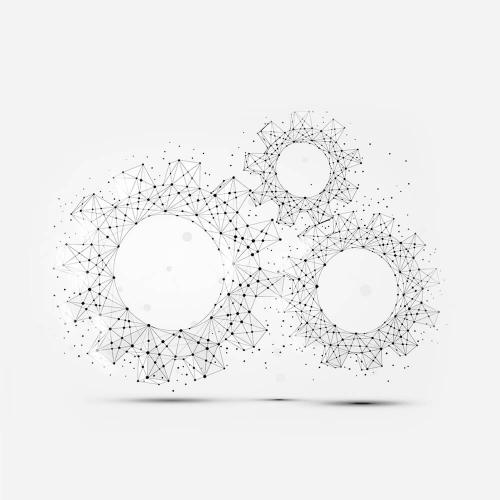
We created this program for those of you, who want to learn how to work with large amounts of information in real-time.
During the first semester, we focus on the essential fundamentals, such as basic concepts of math and programming, which are the same for all programs.
In the Math & Statistic course, students will learn about discrete mathematics and the basics of probability theory. Algorithms & Data Structures covers the basic algorithms on graphs, data structures, and recurrence relations. Also, during the first semester, students learn how to process and analyze data, and build simple models based on this data in the Data Science course. In the Advanced Programming Languages course, students will learn about programming languages and their fundamental differences.
During the second semester, in the Operating Systems course, students will learn about various operating systems and their features. Networks & Clouds course goes over the basics of networking and security. The Systems Architecture course will cover the features of system architecture for different operating systems.
Real-Time Backend is the major course of this program, where students will be offered a real-life project example to work with a large amount of data.
Semester 1
Math & Statistics
Number theory
Sets, functions, and sequences
Logic
Induction
Sequences
Combinatorics
Relations
Recurrence relations
Graphs (basics)
Counting techniques
Graphs and Numbers
Growth of Functions
The probability of an Event
Conditional probability
Discrete Random Variables
Continuous probability
Sampling Theory
Statistics
Algorithms and Data Structures
Graphs (basics)
BFS and DFS
Dynamic Programming
LIS, LCS and other 2D problems
Heaps
Dijkstra, Floyd, Bellman-Ford
DFS applications
Knapsack problem
Dynamic programming over subsets
Amortized Time Complexity (Queue via Stack)
Disjoint Set Union
Dynamic programming on a tree
Minimum Spanning Trees
Matching
Segment Trees
Binary Search Trees
LCA
What is NP and how is it useful?
Strings
Data Science
Basic tools for data analysis
Simple data collection and analysis
Data Types in Statistics
Visualization
Stat in Data Science
Probability and its applications
Estimation, Confidence Intervals
Hypothesis testing
Correlation and Dependence
Regression
Generalized linear model
Feature engineering (Linear Regression Deep Dive)
Decision Trees
ANOVA
Advanced Programming Languages
Grammars, Regular Expressions, Backus-Naur Form
Computation Models
Functional Programming
Clojure Programming Language (Basics, Concurrency, Actors, Refs, Atoms, Macros)
Compiling and linking
Monads
Custom hierarchies and multimethods in Clojure
Logical Programming
Prolog
LLVM (basics, garbage collection)
Constraint Programming
MiniZinc
Guiding the Search in Constraint Programming
Data Oriented Programming
Data storage and querying in XML ecosystem
Semester 2
Networks & Clouds
OSI, analogues, comparison
Data layers of OSI, REST/RPC/SOAP/GraphQL, solutions for this levels
SSH, Telnet, working remotely, solving OverTheWire: Bandit
Media layers
Clouds
Containerization
IoT, smart home, zigbee/bluetooth, wifi, making smart home ourselves
Systems Architecture
C++ - syntax, OOP basics, UB, numbers
RUST - syntax, programming paradigms, safe/unsafe, comparison with C++
Memory allocation, caching algorithms
Memory (its components), cache-friendly algorithms
Assembler (Instructions, RISC, CISC, x86, Intel, asm basics)
Syscalls
Processes, Scheduling
Threads, mutexes, condvars, atomics
Verilog
System limits - rlimit, cgoups, Linux namespaces, seccomp
Real-Time Backend (Architecture)
CAP. MapReduce
Storage. GFS
RPC. Models. Fault tolerance
Physical & logical time, clocks, ordering of events
Broadcast protocols
Consensus and transactions in distributed systems
Election
Consensus
FLP theorem
Raft algorithm
State machine replication
Distributed transactions
Atomic commit protocols
2-phase commit
Distributed File System (DFS)
Industrial Systems Design & System Design. Principles and main concepts. Technology overview
TinyUrl/Pastebin Design Design of industrial systems
Netflix/Youtube architecture
Development of a group project together with a team from the Real-Time Frontend specialty
Operating Systems
UNIX Filesystem basics
Processes and signals
Permissions, ownership, setuid executables
Basics of Bash scripting language
System calls
Usage of system calls in C language
Fork/exec technique
Signal handing in C
Virtual memory
chroot, Linux namespaces and containers
Sockets
Operating system internals